The Python Interpreter¶
Note
Learning objectives: Install the Python interpreter. Use the interpreter as a calculator. Explain what “returning” a value means.
Installing Python¶
Go to the Python Software Foundation web site and download the latest release for your operating system.
Different versions exist for Windows, Linux, MacOS, and others.
- Your not very humble narrator even runs it on his cell phone.
(I need it for good reasons, like breaking into files I stole from your cell phone.)
- You will see two flavors of Python, numbered Python 2 and Python 3.
They are very similar, but Python 3 is more advanced.
Think of Python 2 and Python 3 as separate programming languages.
Make it do math¶
Let’s start our Python interpreter and make it do stuff. Let’s make it do math.
>>> 7 + 5
12
>>> 12 - 5
7
>>> 12 - 5.0 # Now this is weird, right? Where did that decimal point come from?
7.0
>>> 12 / 2
6
>>> 12 / 2.0
6.0
>>> 7 / 2 # I swear I am not making this up. The computer isn't wrong. You are.
3
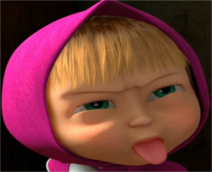
What happens with 7 / 2.0 or 7.0 / 2 ?
Why does it do that?¶
Note
Computer programs read instructions from a disk into memory, then follow those instructions until they end or the computer runs out of resources.
You are running something called the Python interpreter. It’s a program for running other programs.
It takes Python instructions and runs them as soon as you press enter.
Whenever it “thinks” of an answer to something, it shows you right away. That’s called returning a value.
This is not how it will behave when you write a program and run it, but it is a great short-cut to figuring out how it works.
No matter what you give the interpreter, Python will spit back its simplified value.
>>> 5
5
>>> 5.0
5.0
>>> '5'
'5'
Notice how that last '5' is in quotes. I’ll be you thought it was a number. Here’s where the actual fun begins.
Arithmetic operators¶
Here are the math operators for adding, multiplying, getting remainders, etc.
Use this for reference.
Symbol |
Operation |
Result |
Example |
---|---|---|---|
+ |
Add |
Sum |
2 + 5 = 7 |
- |
Subtract |
Difference |
7 - 5 = 2 |
* |
Multiply by |
Product |
2 * 4 = 8 |
/ |
Divide by |
Quotient |
8 / 4 = 2 |
** |
To the power of |
Power value |
2 ** 3 = 8 |
% |
Modulus |
Remainder |
14 % 6 = 2 |
Try some order-of-operations experiments. Go crazy. Use some parentheses.
In fact, use all the parentheses! No one is watching you.
>>> # What will this produce?
>>> 5 - 2 * (16 / 2 - 4) + (2 + 3)**2
>>> # What about this?
>>> 5 - (2 * (16 / 2 - 4) + (2 + 3)**2)
>>> # Unlike teenagers, computers always do what you say.
>>> # ...unless it's after a pound sign.
>>> # A pound sign tells Python to ignore everything after it.
>>> print('I will do this.') # ...but I will not do that.
I will do this.
The “pound sign” (#) is also called a “number sign” or an “octothorpe.”
- Before you even ask: Nope, it is not also called a “hash tag” in computer science.
It might be soon, but there is a story to this. It involves phones, the Olympics and some drama.
Some people are weirdly passionate about their pet octothorpes.