Python Objects and a Simple Web Server¶
Note
Learning objectives: Explain the three aspects of object orientation. Start a basic Python web server and explain how it works. Prove comprehension by overwriting the GET method.
Objects¶
Objects are a complex data structure that has three attributes: encapsulation, inheritance, and polymorphism.
(These will appear on a test in high school – I guarantee it.)
Encapsulation
An object is a container for attributes and methods (functions).
For example, let’s say I have a class called animal with class animal:…
I create an object of that class called Spot with Spot = animal() .
Spot is now an object of type animal(). This is called instantiation. Say it with me.
I set Spot.legs = 4 and Spot now has an attribute called legs which is a variable containing the integer 4.
>>> class animal:
... pass
...
>>> Spot = animal()
>>> Spot.legs = 4
>>> Spot.legs
4
>>> Spot.eyes
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'animal' object has no attribute 'eyes'
Inheritance
We can compose objects of other objects.
Let’s say I create a class called dog which inherits animal with class dog(animal):… .
Now dog has all of the attributes of animal. We say dog is a child class of animal.
1class animal:
2 # We will set some animal defaults
3 legs = 4
4 eyes = 2
5 claws = False
6 tentacles = False
7
8class dog(animal):
9 # Even though you can't see it, dog also has legs
10 # ... and eyes and a few more attributes.
11 tails = 1
12 claws = True
13 adorable = True
14 name = ''
15
16Spot = dog # We instantiate Spot, an object of class dog
17print(Spot.legs)
18print(Spot.eyes)
19print(Spot.tails)
Polymorphism
The language doesn’t care where it gets a name for an attribute or method.
In this example, we have a function called self.istasty() .
It does something different for dog() than it does for the parent class animal().
1class animal:
2 # We will set some animal defaults
3 legs = 4
4 eyes = 2
5 claws = False
6 tentacles = False
7 name = ''
8 edible = True # We assume it's edible
9 def __init__(self, newname=''):
10 if newname:
11 self.setname(newname)
12 while not self.name:
13 self.setname(input('Name this beast: '))
14 def setname(self, newname):
15 self.name = str(newname)
16 def istasty(self):
17 if self.edible:
18 print("%s is edible. Use BBQ sauce!" % self.name)
19 else:
20 print("Do not eat %s." % self.name)
21
22class dog(animal):
23 # Even though you can't see it, dog also has legs
24 # ... and eyes and a few more attributes.
25 tails = 1
26 claws = True
27 adorable = False
28 edible = False
29 name = ''
30 def __init__(self, newname=''):
31 if newname:
32 self.setname(newname)
33 while not self.name:
34 self.setname(input('Name this doggie: '))
35 def istasty(self):
36 if self.edible and not self.adorable:
37 print("%s is edible. Use BBQ sauce!" % self.name)
38 elif self.edible and self.adorable:
39 print("%s is edible, but too cute! Do not eat it!" % self.name)
40 else:
41 print("Do not eat %s." % self.name)
42
43Bessy = animal('Bessy')
44Bessy.istasty()
45
46Spot = dog('Spot') # We instantiate Spot, an object of class dog
47Spot.edible = True
48Spot.adorable = True
49Spot.istasty() # Notice how self.istasty() has new instructions
Spot is counting on you to get this right. Don’t let Spot be food.
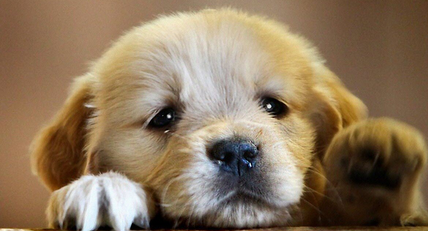
Note
Notice what happens when you just type Spot = dog() . It will make you name your dog.
A Basic Web Server¶
Let’s create a directory from which we will serve our source code.
cd %userprofile%
md serverroot
cd serverroot
Inside that directory, we will place our program, as stolen from https://docs.python.org/3/library/http.server.html
1import http.server
2import os
3import string
4
5# We create an HTTPRequestHandler class that inherits the BaseHTTPRequestHandler
6# ... and lets us play with its attributes and methods
7class HTTPServer_RequestHandler(http.server.BaseHTTPRequestHandler):
8
9 # GET method
10 def do_GET(self):
11 # Send response status code
12 self.send_response(200)
13
14 # Send headers
15 self.send_header('Content-type','text/html')
16 self.end_headers()
17
18 # Send directory listing to the client
19 mydir = string.join(list(os.listdir('.')), '<BR>')
20 message = str('<HTML>' + mydir + '</HTML>')
21 self.wfile.write(bytes(message))
22 return
23
24def run():
25 print('starting server...')
26 server_address = ('', 8008) # a tuple of ip address and port
27 httpd = http.server.HTTPServer(server_address, HTTPServer_RequestHandler)
28 print('Starting!')
29 httpd.serve_forever()
30
31
32run()
For more readings on HTTP methods, such as GET and POST, try https://www.w3schools.com/tags/ref_httpmethods.asp .